For More Info!

Docker: A To Z + Hands On Lab! Part 1
Docker is a powerful tool widely used in DevOps practices to simplify and speed up software development, testing, and deployment. Here’s what Docker is used for in the context of DevOps:
🐳 What is Docker?
Docker is a platform that uses containerization to package applications and their dependencies into a standardized unit—a container—so they can run reliably in any environment.
🚀 How Docker Fits into DevOps Practices
1. Consistent Development Environments
- Containers ensure that the app behaves the same across development, testing, and production.
- Avoids the infamous “works on my machine” problem.
2. Microservices Architecture
- Docker makes it easy to build and manage microservices, where each service runs in its own container.
- Enables scaling and independent updates of parts of an application.
3. Faster Deployment
- Containers start up quickly compared to virtual machines.
- Makes CI/CD pipelines (Continuous Integration/Continuous Deployment) faster and more reliable.
4. Version Control for Environments
- Docker files (used to build images) can be tracked in source control.
- Enables infrastructure-as-code practices.
5. Portability
- Docker containers can run on any system that supports Docker: local dev machines, cloud servers, Kubernetes clusters, etc.
6. Testing and Automation
- Containers are ideal for automated testing: spin up test environments, run tests, destroy the environment.
- Easily integrates into tools like Jenkins, GitLab CI, GitHub Actions, etc.
7. Isolation
- Each container is isolated from the host and other containers.
- Helps with security and dependency management.
🔧 Common DevOps Use Cases
- Building and running CI/CD pipelines
- Creating reproducible development environments
- Running automated tests in isolation
- Deploying containerized applications to Kubernetes or other orchestrators
- Scaling apps in production without downtime
Docker Commands Cheat Sheet
🧩 Why Do We Use Docker?
Docker allows you to run applications in isolated, consistent environments called containers, making development, testing, and deployment faster and easier.

Example:
You have a Windows machine but want to run Ubuntu. Instead of installing a full VM, just use:
docker run -it ubuntu

Note:
Why this error?
This error occurs because the Docker Desktop app is not running.
👉 Make sure to start Docker Desktop first, then run your command.
install and run this app in your OS

Re-run command in Terminal


Can Check/verify also in Docker desktop app


To find Images : Search on google -> Docker Hub Container Image Library | App Containerization
Docker Hub Commands for Terminal Use
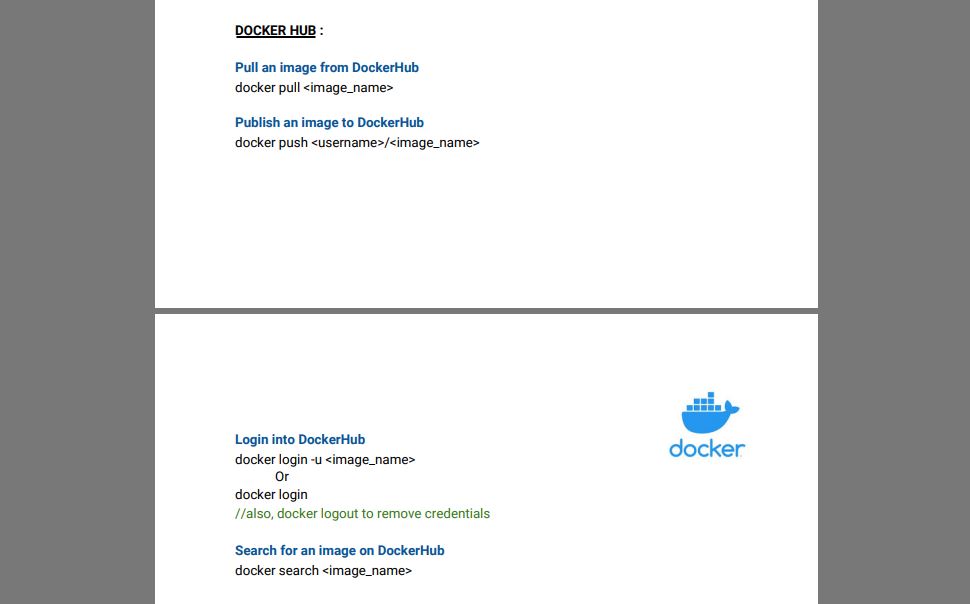
or you simply search images via docker hub web page

Search Any Image : Example hello-world

Shortlisted : These are go-to commands for day-to-day tasks

Open New terminal or write exit in current terminal session and Pull hello-world image
docker pull hello-world



-it (interactive)
Use command with -it ( interactive _ teletype )
When Docker suggests using -it
, it’s pointing to two combined options: -i
and -t
. Here’s what they mean and why they matter:

🔍 -i
→ Interactive
- Stands for interactive mode.
- Keeps STDIN open, even if you’re not attached to the container.
- This is necessary if you want to type commands into the container after it’s started.
🎮 -t
→ TTY (Teletype)
- Allocates a pseudo-TTY (terminal).
- Makes the container behave more like a real terminal (e.g., with color, command editing, etc.).
🧠 So, when you use -it
together:
You’re telling Docker:
“Keep the input open and connect me to a real terminal session inside the container.”
✅ Benefits of -it
- You can interact with the container using a shell like
bash
. - Useful for debugging, exploring, or running commands manually.
- Makes the container feel like you’re SSH’d into a regular server.
🧪 Example:
docker run -it ubuntu
This gives you a bash prompt inside the Ubuntu container — you can now run commands like you’re in a normal Ubuntu machine.
🔁 Versus without -it
:
docker run ubuntu
This runs and exits immediately, unless you’re running a long-running process. No terminal, no interaction.
List
use of Docker PS : List of all running containers ( running and stop)

Start
Use of Docker Start

Stop
There are a few ways to stop a Docker container via the terminal
here’s the quick guide:
✅ 1. If you’re inside the container (using -it
):
Just press:
Ctrl + D
or type:
exit
That will exit the shell, and if it’s the main process, the container will stop.
✅ 2. If you’re outside and want to stop it by name or ID:
Step 1: Find the running container:
docker ps
You’ll see something like:
CONTAINER ID IMAGE COMMAND ... NAMES
a1b2c3d4e5f6 ubuntu "/bin/bash" ... my_container
Step 2: Stop the container:
docker stop <container_id_or_name>
Example:
docker stop a1b2c3d4e5f6
or
docker stop my_container
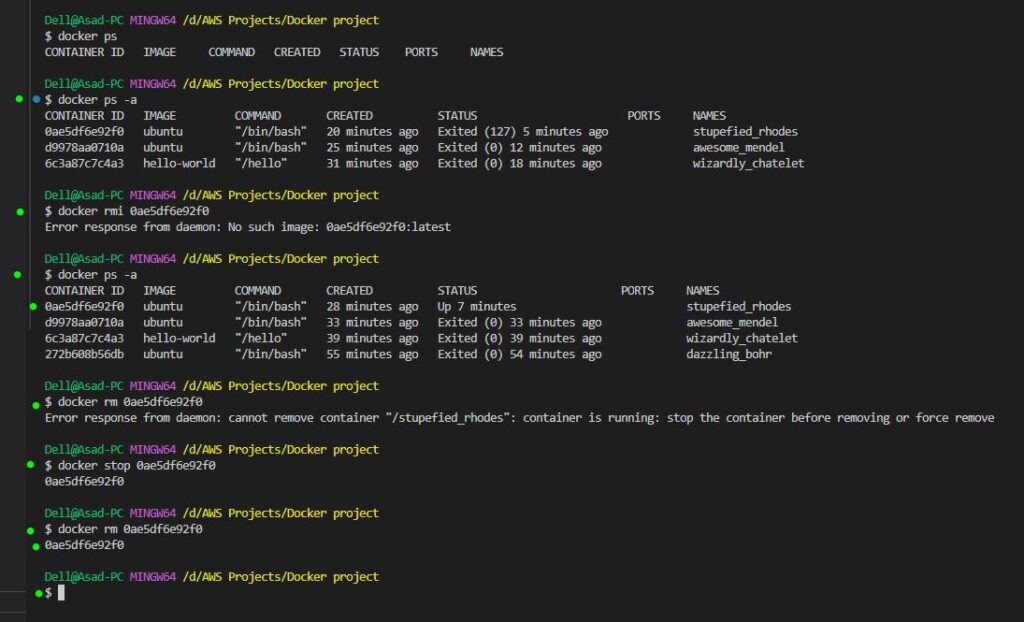
🧹 Bonus: Stop all running containers:
docker stop $(docker ps -q)
Versions

example 1 : pulling mysql LATEST

example 2 : pulling mysql with version specific
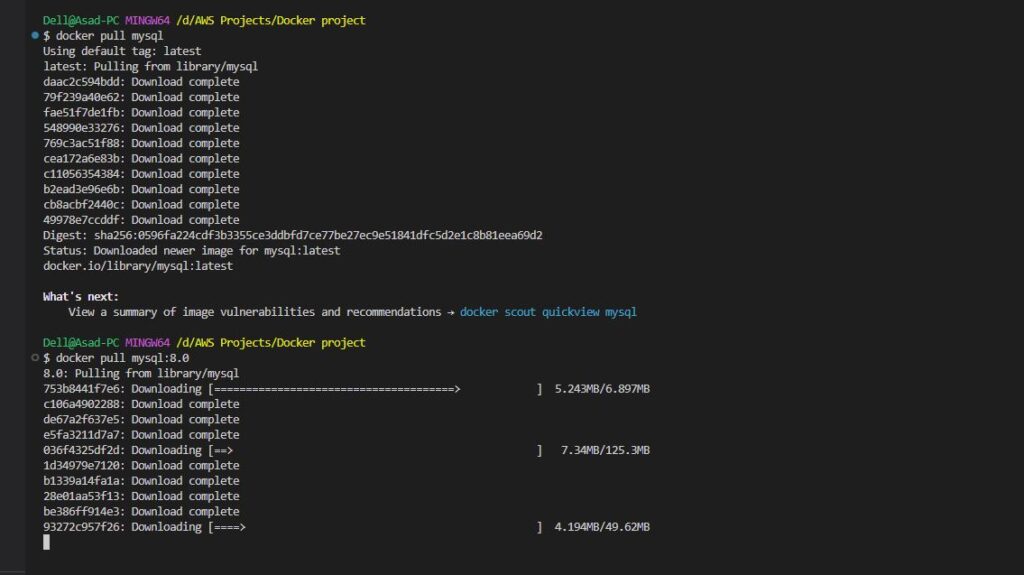
Absolutely! Let’s break each of those down with clear explanations and examples:
🔹 1. Docker Detached Mode (-d
)
👉 What it does:
- Runs the container in the background (detached from your terminal).
- You won’t see logs or interact with it directly in the terminal.
📦 Example:
docker run -d ubuntu sleep 60
This runs the Ubuntu container, sleeps for 60 seconds in the background.
✅ Why use it?
- You want the container to keep running while your terminal stays free.
- Great for running servers (like NGINX, MySQL, etc.)
🔹 2. Docker “latest” Image Tag
👉 What it means:
- If you don’t specify a version, Docker uses the image tagged as
latest
.
📦 Example:
docker run ubuntu
is the same as:
docker run ubuntu:latest
But “latest” doesn’t always mean the newest version — it just means the image that the maintainer tagged as latest
. So it’s not always safe in production.
🔹 3. Docker with Specific Version
👉 Why use it?
- To avoid surprises when the base image updates and breaks your app.
📦 Example:
docker run ubuntu:20.04
This ensures you’re using Ubuntu 20.04, not the latest or whatever is tagged latest
.
You can check available versions here: https://hub.docker.com/_/ubuntu
🔹 4. docker run --name CONTAINER_NAME -d IMAGE_NAME
👉 What it does:
- Runs a container in detached mode
- Names the container (so you can reference it easily)
- Uses a specific image
📦 Example:
docker run --name my_web -d nginx
This will:
- Run an NGINX web server
- In the background
- With the container name
my_web
You can then stop it easily:
docker stop my_web

✅ Goal
Run a MySQL container with:
- A custom name
- Root password
- Detached mode
- MySQL image
💥 Error:
docker: invalid reference format: repository name (library/IMAGE_NAME) must be lowercase.
🔍 You ran:
docker run --name CONTAINER_NAME -d IMAGE_NAME
But IMAGE_NAME
is just a placeholder — not an actual image. Docker needs a real lowercase image name (like mysql
).
✅ Correct Example
docker run --name some-mysql -e MYSQL_ROOT_PASSWORD=my-secret-pw -d mysql
--name some-mysql
→ custom name-e MYSQL_ROOT_PASSWORD=...
→ required by MySQL-d
→ detached modemysql
→ actual image
📌 For specific version:
docker run --name some-mysql -e MYSQL_ROOT_PASSWORD=my-secret-pw -d mysql:8.0
🧪 What You Did:
docker run -d mysql
✅ Worked — but no root password, so MySQL may error.
docker run -d -e MYSQL_ROOT_PASSWORD=secret mysql
✅ Better — password is provided, but no name given (Docker auto-named it).
✅ Best Practice (Recommended)
docker run --name my-mysql -e MYSQL_ROOT_PASSWORD=secret -p 3306:3306 -d mysql:8.0
- Easy to manage with
--name
- Root password set
- Exposes MySQL port
- Specific version = stable builds
Real-time
Realtime Scenario in Vs code bash terminal

Here’s a short explanation of what happened:
🧱 1. You ran a MySQL container:
docker run -d -e MYSQL_ROOT_PASSWORD=secret mysql
- Started a MySQL container in the background.
- Docker auto-named it:
heuristic_diffie
.
🔍 2. You checked running containers:
docker ps
- Shows your MySQL container is running, with default ports.
📦 3. You ran another MySQL container with a name + version:
docker run -d -e MYSQL_ROOT_PASSWORD=secret --name mysql-older mysql:8.0
- Started another MySQL container.
- Named it
mysql-older
, and used MySQL version 8.0.
✅ 4. docker ps
shows both running containers:
mysql-older
(version 8.0, named manually)heuristic_diffie
(latest version, auto-named)
Layers

🔹 What are Docker Layers?
Docker image layers are like building blocks stacked on top of each other.
From bottom to top:
- Base Layer – The foundation (e.g., Ubuntu, Alpine).
- Layer 1, Layer 2 – Added by each instruction in your Dockerfile (like
RUN
,COPY
, etc.). - Container – The top layer that’s writable, where your running app lives.
🧠 Why Layers Matter?
- ✅ Reusability – Shared across images = faster builds.
- ✅ Caching – Only changed layers are rebuilt.
- ✅ Efficiency – Smaller, modular image sizes.
Latest, older versions comparisons

🧾 In Short:
- You pulled
mysql:latest
, but already hadmysql:8.0
. - Docker reuses common layers → shows
already exists
. - Only new layers get downloaded.
- ✅ Saves time, space, speed up pulls, and bandwidth.

Port Binding



🧾 Docker Port Binding:
- Port binding allows you to map a container’s internal port to a port on your host machine.
- This enables you to access services running inside a container (like a web server or database).
🔑 Syntax:
docker run -p <host_port>:<container_port> <image_name>
🔹 Example 1: Exposing a web server
docker run -d -p 8080:80 nginx
- Host: Port
8080
→ Container: Port80
(NGINX default). - Access the server via
http://localhost:8080
.
🔹 Example 2: Database (MySQL)
docker run -d -p 5000:3306 mysql
- Host: Port 5000 → Container: Port
3306
(MySQL default).
✅ Why use it?
- To connect external apps (or your browser) to the services inside containers.
- Can bind multiple ports or different host ports.