For More Info!
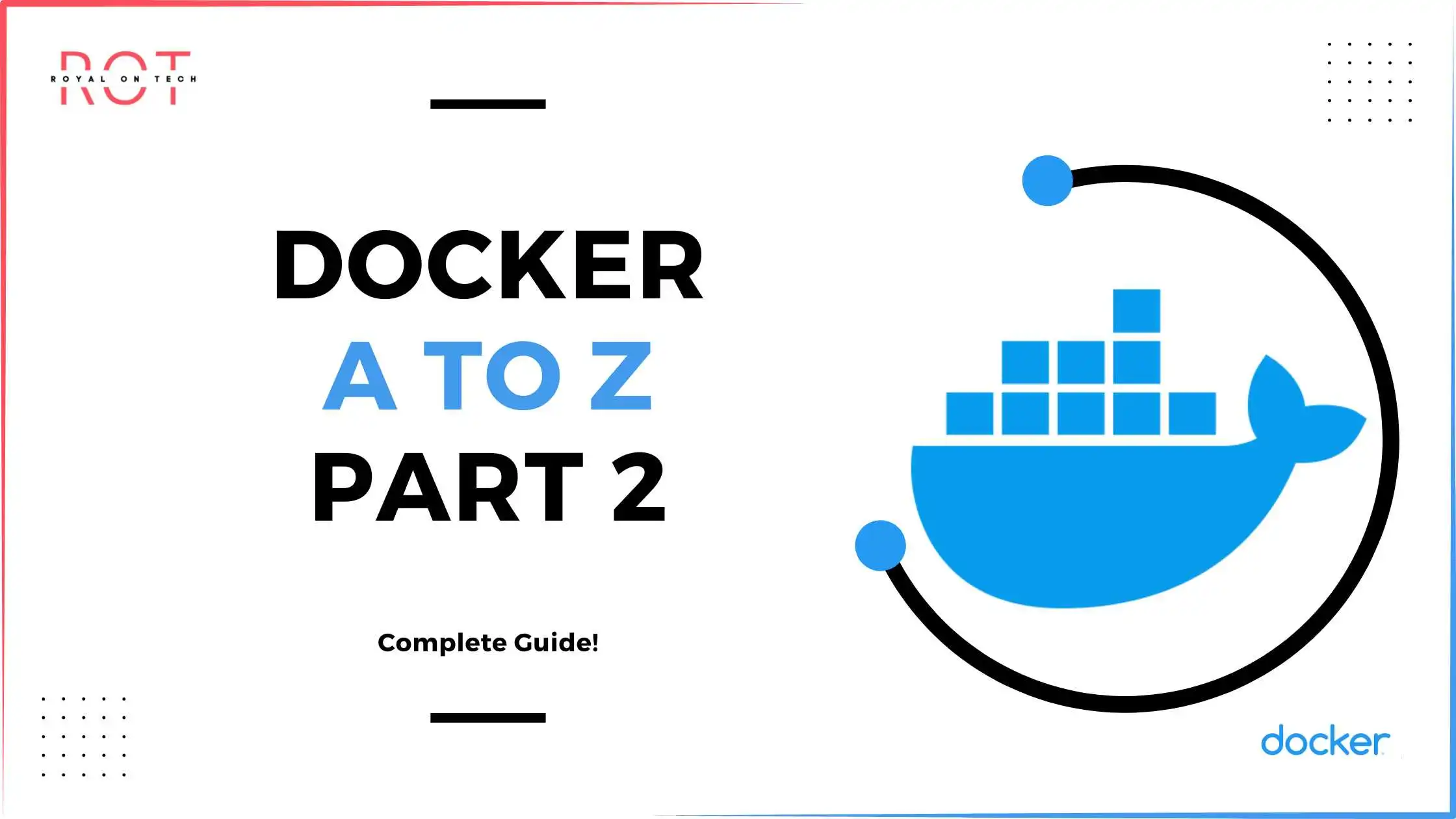
Docker: A To Z + Hands On Lab! Part 2
Troubleshooting
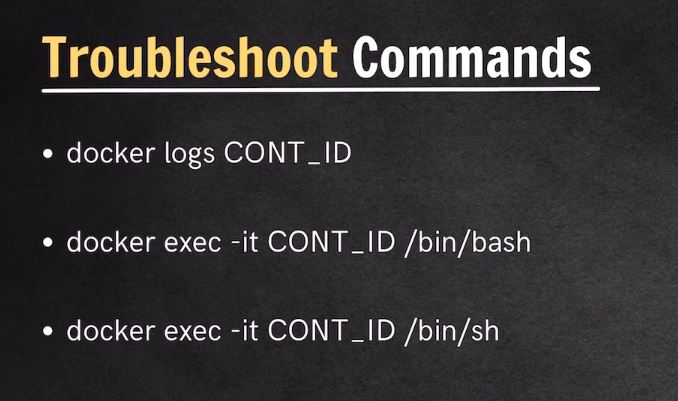
1 – 🧾 Docker Logs (In Short)
Docker logs let you see the output (stdout/stderr) from a running container — like error messages, server output, etc.
🔹 Basic Command:
docker logs <container_name_or_id>
🔹 Examples:
docker logs my-mysql
Shows logs from the my-mysql
container.
🔧 Useful Options:
-f
→ Follow logs (liketail -f
)docker logs -f my-app
--tail
→ Show last N linesdocker logs --tail 50 my-app
- Combine both:
docker logs -f --tail 100 my-app
✅ Use Case:
- Debugging errors
- Checking service output
- Monitoring container activity
View logs on VS code terminal

View logs on Docker Desktops

2nd
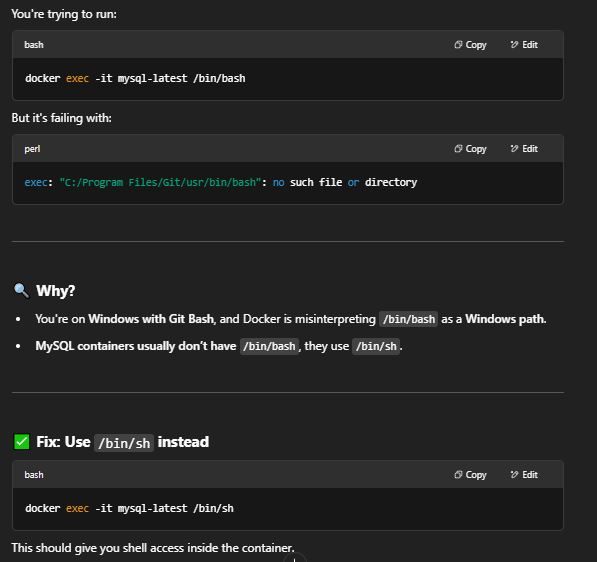
exec errors Logs – Use Wimpty
You’re on Git Bash + Windows, and it’s messing up the path.

Git Bash is rewriting /bin/sh
or /bin/bash
into a Windows path like:
C:/Program Files/Git/usr/bin/sh
which Docker doesn’t understand.
If Not Then try this below
✅ Fix (Use winpty)

Run the command with winpty
in Git Bash:
winpty docker exec -it mysql-latest sh
If that doesn’t work, try:
winpty docker exec -it mysql-latest bash
🧠 Why it works:
winpty
fixes the interactive terminal handling on Windows + Git Bash.
Docker Vs VM

🧾 Docker vs Virtual Machine (VM) — In Short
Feature | Docker (Containers) | Virtual Machines (VMs) |
---|---|---|
Speed | 🏎️ Fast startup (~seconds) | 🐢 Slower (~minutes) |
Lightweight | ✅ Yes (shares OS kernel) | ❌ Heavy (full OS per VM) |
Resources | Uses less CPU/RAM | Uses more CPU/RAM |
Isolation | Process-level isolation | Full OS-level isolation |
Portability | High (same image runs anywhere) | Medium (depends on OS/Hypervisor) |
Boot OS? | ❌ No (uses host OS kernel) | ✅ Yes (each VM has its own OS) |
✅ Docker Use Case
- Microservices
- Dev/testing environments
- Lightweight apps
✅ VM Use Case
- Run different OS (e.g., Linux on Windows)
- Full system simulation
- Legacy apps requiring full OS
💡 TL;DR:
Docker = Lightweight, fast, app-level.
VM = Heavy, full OS, better isolation.
Note : Docker relies on the Docker Desktop application to run images and containers on Windows and macOS systems. Thats why we use this softwere on windows and MacOS
🧾 Docker Desktop = Combo of Tools + VM (on Windows/macOS)

🧠 On Windows/macOS, Docker Desktop:
- Runs a lightweight VM behind the scenes using Hyper-V (Windows) or Apple Hypervisor/VirtualBox (macOS)
- This VM runs Linux, where Docker Engine lives
- So yes — it acts like a hypervisor to enable Docker on non-Linux systems
💡 So:
Platform | How Docker Runs |
---|---|
Linux | Native (no VM needed) |
Windows/macOS | Via Docker Desktop → Runs Linux VM |
✅ Why This Matters:
- On Windows/macOS, Docker Desktop is essential to simulate a Linux environment (since Docker uses Linux kernel features like cgroups & namespaces).
- Docker CLI talks to the Linux VM in the background.
Dev With Docker
Network
🧾 What is Docker Network?
Docker networking lets containers talk to each other, and optionally to the outside world (your host, internet, etc.).

🔌 Types of Docker Networks:
Network Type | Description |
---|---|
bridge (default) | Default network for containers; containers can talk to each other using container names. |
host | Container shares the host’s network (no isolation). Faster, but less secure. |
none | No networking at all (totally isolated). |
custom bridge | Like bridge, but you create and control it. Enables easier name-based communication between containers. |
overlay | For multi-host Docker (Swarm); allows containers across machines to communicate. |
🧪 Example: Custom Network
docker network create my-net
Then run two containers:
docker run -d --name app1 --network my-net nginx
docker run -d --name app2 --network my-net alpine sleep 9999
Now app2
can ping app1
:
docker exec -it app2 ping app1
✅ It works because they share the same network.
🎯 Use Cases:
- Link app and database containers
- Control traffic between services
- Enable service discovery by container name
DB Setup
Setting up multiple containers via terminal gets messy—port bindings, environment variables, custom names—it’s easy to make mistakes.
Setting up mongo and mongo-express via docker command in terminal
(complicated + not recommended)

type this in your terminal to setup mongo database
docker run -d \
-p27017:27017 \
--name mongo \
--network mongo-network \
-e MONGO_INITDB_ROOT_USERNAME: admin \
-e MONGO_INITDB_ROOT_PASSWORD: qwerty \
mongo




DOCKER COMPOSE (recommended method ) .yaml
yet another markup language = yaml
Why Use Docker Compose?
Docker Compose solves this by letting you define everything in a single .yml
file. It’s a tool for managing multi-container apps in a structured, easy-to-read way.
✅ Clean and organized
✅ Easy to edit and reuse
✅ No more long terminal commands
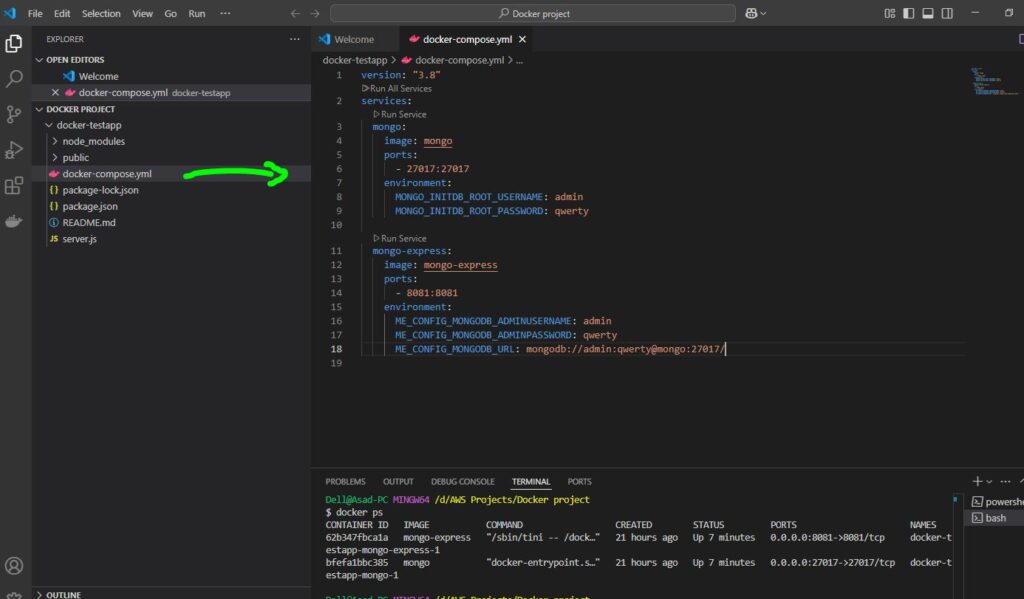

Just run docker-compose up
and you’re good to go!
or run commands in image shown!
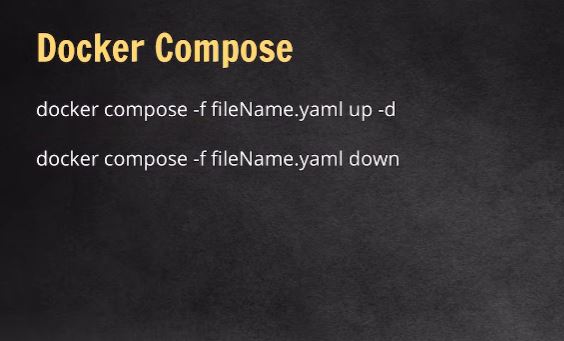
Docker command Vs Docker Compose

✅ .yaml
vs .yml


There is no difference between .yaml
and .yml
— both are valid YAML file extensions.
📝 Why two options?
.yml
existed because early systems (like Windows) had issues with 4-letter extensions..yaml
is the official extension (as per YAML spec).
👍 Use either:
.yaml
is preferred in modern tools and docs.- But Docker, GitHub Actions, etc., support both.
✅ Step-by-Step Verification of MongoDB via Mongo Express

1. Start Your Containers
Make sure you’re in the directory where your docker-compose.yml
file is, then run:
docker-compose up -d
This will start:
mongo
(your database)mongo-express
(your web UI)
2. Visit Mongo Express in Browser
Go to:
👉 http://localhost:8081
You should see something like this:

🔒 Login Prompt
Mongo Express will auto-login if the environment variables are correct.
If it prompts for username/password, it uses the values from your docker-compose.yml
:
ME_CONFIG_MONGODB_ADMINUSERNAME: admin
ME_CONFIG_MONGODB_ADMINPASSWORD: qwerty
So use:
- Username:
admin
- Password:
qwerty
or pass
📸 Sample Screenshots (Visual Guide)

1. Mongo Express Dashboard
You should see:
- Your MongoDB server connected
- List of databases (e.g.,
admin
,local
) - Options to view collections, insert documents, etc.
2. If Login Fails
If it fails to connect:
- Make sure
mongo
andmongo-express
are in the same Docker network (which they are by default indocker-compose
) - Double-check your
docker-compose.yml
indentation (YAML is picky!) - Ensure you’re using correct ports:
8081
on your host, mapped to8081
in the container
Fix This : http://localhost:5050/getUsers
its for to verify database entries
🛑 To Stop & Remove Containers from a YAML File
From the same directory where your docker-compose.yml
lives, run:
docker-compose down
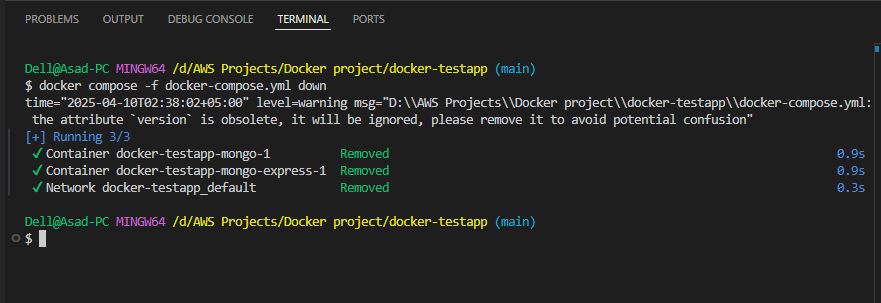
This will:
- Stop all containers started with
docker-compose up
- Remove those containers
- But it won’t delete volumes or images unless you ask it to
Optional Flags:
🔥 To Also Remove Volumes (databases/data):
docker-compose down -v
🧼 To Also Remove Images:
docker-compose down --rmi all
📁 If You’re Asking How to “Download” a YAML File
If you meant download a sample YAML file, here’s a simple one you can copy and save as docker-compose.yml
:

version: "3.8"
services:
mongo:
image: mongo
ports:
- "27017:27017"
environment:
MONGO_INITDB_ROOT_USERNAME: admin
MONGO_INITDB_ROOT_PASSWORD: qwerty
mongo-express:
image: mongo-express
ports:
- "8081:8081"
environment:
ME_CONFIG_MONGODB_ADMINUSERNAME: admin
ME_CONFIG_MONGODB_ADMINPASSWORD: qwerty
ME_CONFIG_MONGODB_URL: mongodb://admin:qwerty@mongo:27017/
Save this in your project folder as docker-compose.yml
.